
Table of Contents
In the previous section, we established the importance of navigation and discussed the fundamentals of setting up React Navigation. As we dive further into this guide, we will now explore one of the fundamental components of mobile application navigation - the Stack Navigation. This section will take you through the anatomy of a stack navigator, illustrating the ease of screen transitions and the method to transmit parameters between screens. We hope this section clears the path for you to construct an efficient stack navigation structure for your application. Let's dive in!
Building a Gatsby Blog Series
The anatomy of a stack navigator: set-up and route initialization
The first step to create a stack navigation is to install the stack navigator package:
npm install @react-navigation/stack
Then, create a stack navigator and define initial routes. Routes are defined using the Screen component, and the navigator should wrap all Screen components.
import { createStackNavigator } from '@react-navigation/stack';
const Stack = createStackNavigator();
function MyStack() { return ( <Stack.Navigator initialRouteName="Home"> <Stack.Screen name="Home" component={HomeScreen} /> <Stack.Screen name="Details" component={DetailsScreen} /> </Stack.Navigator> );}
The art of screen transition using stack navigation
You can navigate to different screens using the navigation.navigate function provided in your screen component's props.
function HomeScreen({ navigation }) { return ( <View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}> <Button title="Go to Details" onPress={() => navigation.navigate('Details')} /> </View> );}
The practice of parameter transmission between screens
You can pass parameters while navigating to screens. These parameters can be accessed through the route prop in the target screen.
function HomeScreen({ navigation }) { return ( <Button title="Go to Details" onPress={() => navigation.navigate('Details', { itemId: 86 })} /> );}
function DetailsScreen({ route }) { const { itemId } = route.params;
return <Text>Item Id: {JSON.stringify(itemId)}</Text>;}
FAQ
- What is the initialRouteName prop in Stack.Navigator?
The initialRouteName prop determines which screen should be shown first when the app loads.
- What does navigation.navigate do?
The navigation.navigate function allows you to navigate to different screens. You can pass the name of the route you want to navigate to as an argument.
- How do I pass parameters to a screen during navigation?
You can pass parameters as a second argument to the navigation.navigate function. These parameters will be available in the route.params object in the target screen.
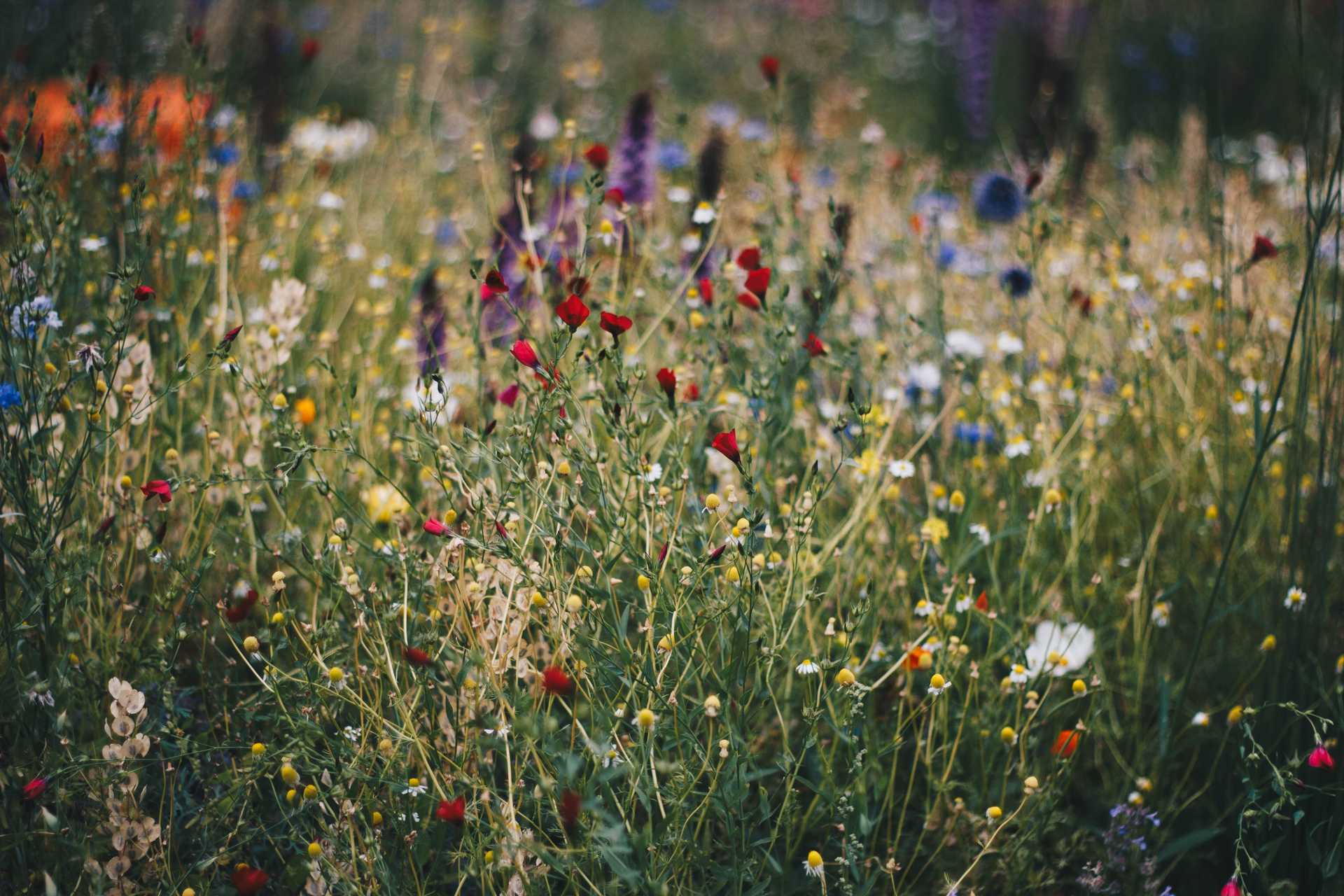

Navigation is an integral part of any mobile application, providing a pathway for users to traverse various screens and interfaces. React Navigation, a popular…