
Table of Contents
As we move further into the intricacies of navigation in React Native applications, we encounter several unique patterns that address specific needs. From the implementation of authentication flows to bottom tab navigation with stack screens and conditional navigation, these patterns serve to create a more dynamic and interactive user experience. In this section, we will delve into these patterns, providing examples and explanations to guide your understanding. By the end, you'll have a deeper grasp of the versatility and potential of React Navigation.
Building a Gatsby Blog Series
Authentication flow: Implementing login and signup screens
You can create an authentication flow using a stack navigator. Here's an example of how you can do it:
<Stack.Navigator> {isLoggedIn ? ( <> <Stack.Screen name="Home" component={HomeScreen} /> <Stack.Screen name="Profile" component={ProfileScreen} /> {/* other logged in screens */} </> ) : ( <> <Stack.Screen name="SignIn" component={SignInScreen} /> <Stack.Screen name="SignUp" component={SignUpScreen} /> {/* other logged out screens */} </> )}</Stack.Navigator>
Bottom tab navigation with stack screens
You can create a tab navigator with each tab containing a stack of screens:
<Tab.Navigator> <Tab.Screen name="Home" component={HomeStackScreen} /> <Tab.Screen name="Settings" component={SettingsStackScreen} /></Tab.Navigator>
Conditional navigation: Navigating based on certain conditions
You can navigate conditionally based on certain state or props:
function ProfileButton({ navigation, user }) { return ( <Button title="Open profile" onPress={() => user ? navigation.navigate('Profile', { user }) : navigation.navigate('SignIn') } /> );}
FAQ
- How can I implement an authentication flow in my application?
You can implement an authentication flow by using a stack navigator and conditionally rendering different sets of screens based on whether the user is logged in.
- How can I have each tab in a tab navigator contain a stack of screens?
You can have each tab contain a stack of screens by making each Screen component in your Tab.Navigator a stack navigator.
- How can I navigate conditionally based on certain conditions?
You can navigate conditionally by calling navigation.navigate with different screen names based on your application's state or props.
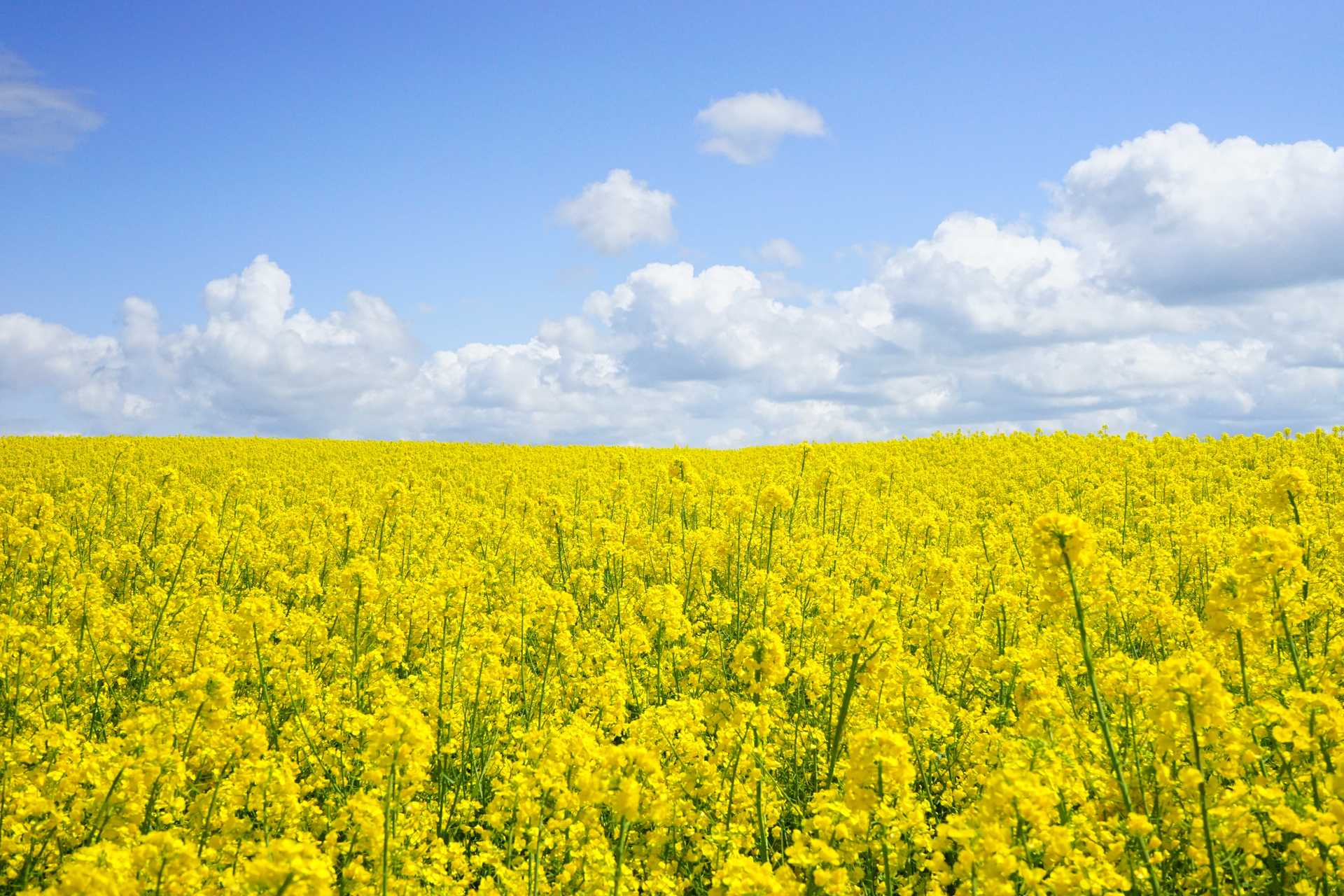

In any application, the ability to customize the look and feel of navigation components is essential. It not only enhances the user experience but also ensures…